firebase 推送
Ever wondered how does your smartphone receive and handle notifications whether be in foreground, background or even killed?
有没有想过您的智能手机如何接收和处理处于前台,后台甚至被杀死的通知?
Firebase Cloud Messaging is the tool used to send push notifications to single or a group of devices.
Firebase Cloud Messaging是用于将推送通知发送到单个或一组设备的工具。
Firebase Cloud Messaging: Firebase Cloud Messaging (FCM) is a cross-platform messaging solution that lets you reliably send messages at no cost.
Firebase云消息传递: Firebase Cloud Messaging(FCM)是一种跨平台的消息传递解决方案,可让您可靠地免费发送消息。
Using FCM, you can notify a client app that new email or other data is available to sync. You can send notification messages to drive user re-engagement and retention. For use cases such as instant messaging, a message can transfer a payload of up to 4KB to a client app.
使用FCM,您可以通知客户端应用程序可以同步新电子邮件或其他数据。 您可以发送通知消息来推动用户的重新参与和保留。 对于即时消息之类的用例,一条消息可以将最多4KB的有效负载传输到客户端应用程序。
Now, as you have got a little idea about FCM, let’s implement it to our Android Studio Project.
现在,您对FCM有了一些了解,让我们将其实现到我们的Android Studio项目中。
Create Firebase Project:
创建Firebase项目:
Go to Firebase Console.
转到Firebase 控制台 。
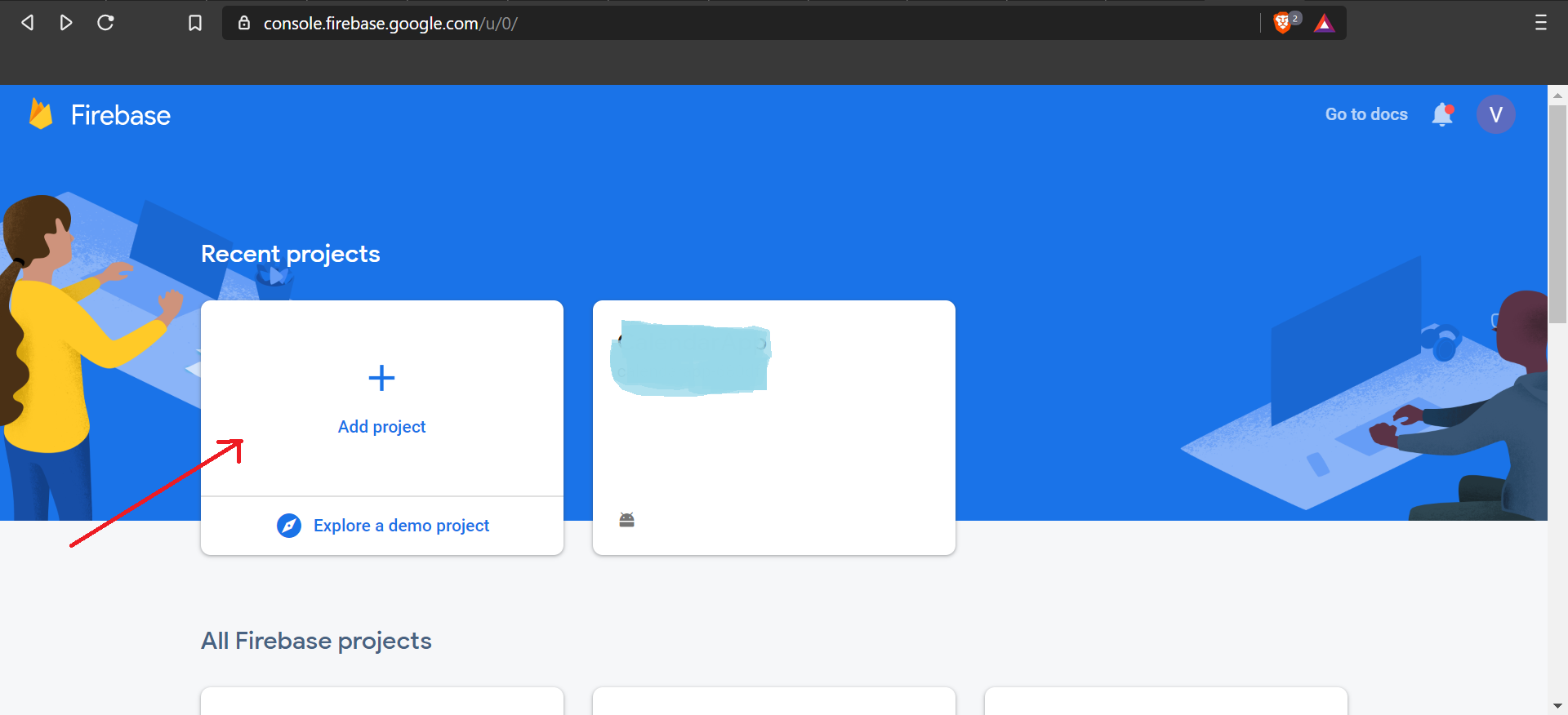
Follow the steps to set up the project.
请按照以下步骤设置项目。
After adding project to the Firebase, add App to the same project.
将项目添加到Firebase后,将App添加到同一项目。
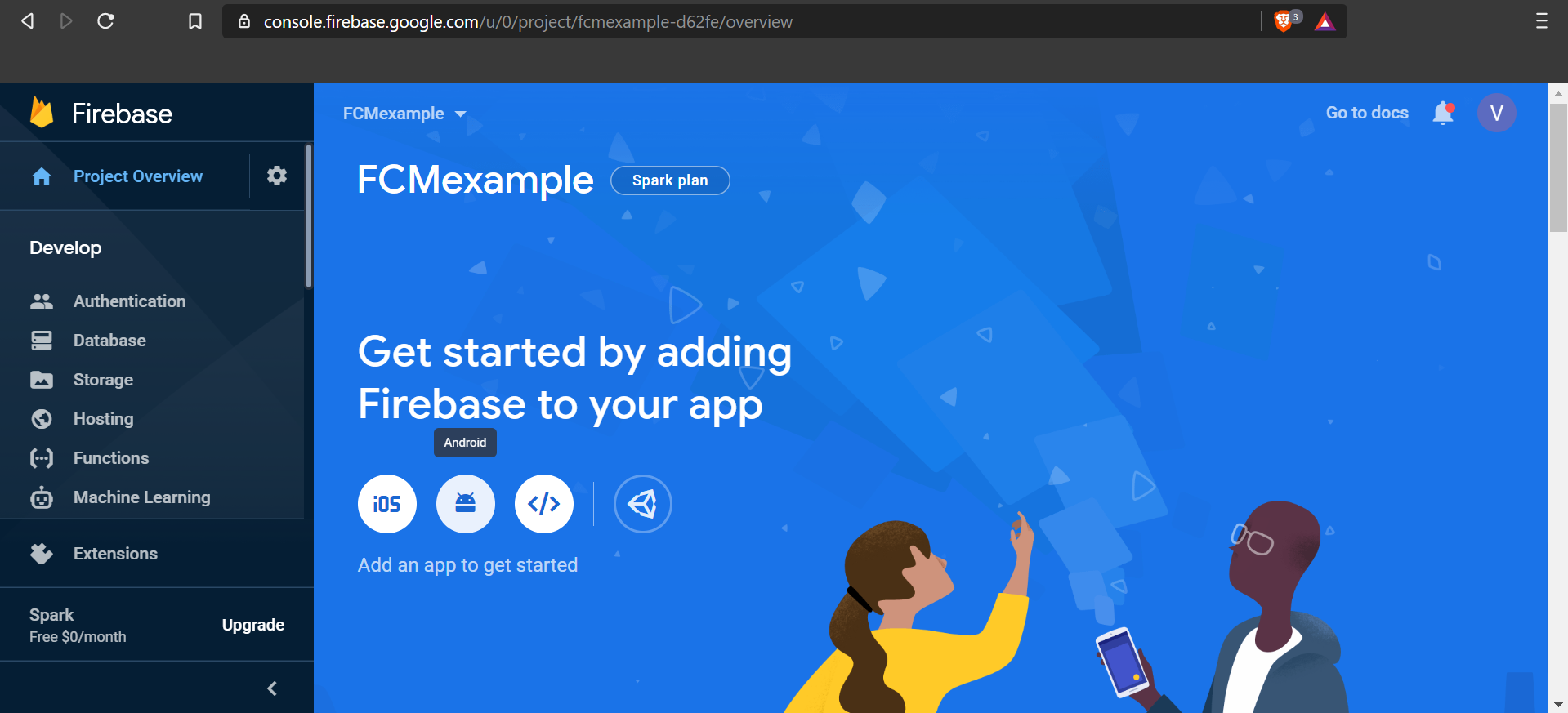
Enter the project name, package name and SHA-1 key of your Android Studio project.
输入您的Android Studio项目的项目名称,程序包名称和SHA-1键。
Follow all the steps to complete the Android App linking with Firebase.
请按照所有步骤完成与Firebase的Android应用链接。
If you face any problem while setting up Firebase on your project you can refer to Add Firebase to your Android Project.
如果在项目上设置Firebase时遇到任何问题,可以参考将Firebase添加到Android项目。
Till now, we have only linked our Android Studio project to Firebase. Now, we proceed with adding Firebase SDK to our project to handle FCM.
到目前为止,我们仅将Android Studio项目链接到Firebase。 现在,我们将Firebase SDK添加到我们的项目中以处理FCM。
添加Firebase SDK: (Adding Firebase SDK:)
Step 1: Add below code into <project>/build.gradle file.
步骤1:将以下代码添加到<project> /build.gradle文件中。
buildscript {
dependencies {
// Add this line
classpath 'com.google.gms:google-services:4.3.2'
}
}
Step 2: Add below code into <project>/<app>/build.gradle.
步骤2:将以下代码添加到<project> / <app> /build.gradle中。
dependencies {
// Add this line
implementation 'com.google.firebase:firebase-messaging:20.0.0'
}
...
// Add to the bottom of the file
apply plugin: 'com.google.gms.google-services'
Step 3: Now, press Sync Now to sync the changes in gradle.
第3步:现在,按立即同步以同步Gradle中的更改。
Since, the formalities are done we are now into some real stuff.
既然手续已经完成,我们现在就涉及一些实际内容。
Message Targeting Types:
邮件定位类型:
Single Device: To send FCM message to a single device we need to know the Registration Token of the client app which is generated by Firebase SDK. This token is dynamic and keeps on changing after some time.
单个设备:要将FCM消息发送到单个设备,我们需要知道由Firebase SDK生成的客户端应用程序的注册令牌。 该令牌是动态的,一段时间后会不断变化。
How to get current Registration Token?
如何获得当前的注册令牌?
FirebaseInstanceId.getInstance().getInstanceId()
.addOnCompleteListener(new OnCompleteListener<InstanceIdResult>() {
@Override
public void onComplete(@NonNull Task<InstanceIdResult> task) {
if (!task.isSuccessful()) {
Log.w(TAG, "getInstanceId failed",
task.getException());
return;
}
// Get new Instance ID token
String token = task.getResult().getToken();
}
});
2. Group of devices: To send FCM message to a group of devices, all such devices need to subscribe to a common topic named “xyz”.
2. 设备组:要向一组设备发送FCM消息,所有此类设备都需要订阅一个名为“ xyz”的通用主题。
What is Topic and How to subscribe to it:
什么是主题以及如何订阅:
Topic is like a mailing list, where devices belonging to a common topic receive a common particular message.
主题就像一个邮件列表,其中属于某个公共主题的设备会收到一条公共的特定消息。
Paste the following code in the Activity to subscribe or unsubscribe.
将以下代码粘贴到活动中以进行订阅或取消订阅。
Subscribe Topic :FirebaseMessaging.getInstance().subscribeToTopic("TopicName");Unsubscribe Topic : FirebaseMessaging.getInstance().unsubscribeFromTopic("TopicName");
Retain this for some time as I would now give you glimpse about the type of Firebase Messages.
将其保留一段时间,因为我现在将简要介绍一下Firebase消息的类型。
Types of Firebase Messages:
Firebase消息的类型:
Notification Payload: Notification messages provide nothing much for customization and have fixed object key values( would get to know about it later ) : “title” and “body”.
通知有效负载:通知消息没有提供任何自定义功能,并且具有固定的对象键值(稍后会了解):“标题”和“正文”。
{
"message":{
"token":"bk3RNwTe3H0:CI2k_HHwgIpoDKCIZvvDMExUdFQ3P1...",
"notification":{
"title":"Portugal vs. Denmark",
"body":"great match!"
}
}
}
2. Data Payload: Data messages have to be handled by the android app. You can add this kind of messages if you want to send some only data along with the notification. It contains custom key value pairs.
2.数据有效负载:数据消息必须由android应用处理。 如果您只想发送一些数据和通知,则可以添加此类消息。 它包含自定义键值对。
{
"message":{
"token":"bk3RNwTe3H0:CI2k_HHwgIpoDKCIZvvDMExUdFQ3P1...",
"data":{
"Nick" : "Mario",
"body" : "great match!",
"Room" : "PortugalVSDenmark"
}
}
}
3. Bothof the above two: A message can also contain both notification and data payload. When these kind of messages are sent, it will be handled in two scenarios depending upon app state (background / foreground). For these message we can use both notification and data keys.
3.以上两者:消息还可以同时包含通知和数据有效负载。 发送此类消息后,将根据应用程序状态(后台/前台)在两种情况下进行处理。 对于这些消息,我们可以同时使用通知键和数据键。
{
"message":{
"token":"bk3RNwTe3H0:CI2k_HHwgIpoDKCIZvvDMExUdFQ3P1...",
"notification":{
"title":"Portugal vs. Denmark",
"body":"great match!"
},
"data" : {
"Nick" : "Mario",
"Room" : "PortugalVSDenmark"
}
}
}
处理Firebase消息: (Handling Firebase Messages:)
Handling firebase messages is the most crucial part of this whole blog.
处理Firebase消息是整个博客中最关键的部分。
Basically, FCM messages are handled differently depending upon the Payload type and the state of the app i.e Foreground, Background or Killed.
基本上,FCM消息的处理方式取决于有效负载类型和应用程序的状态(例如,前台,后台或已终止)。
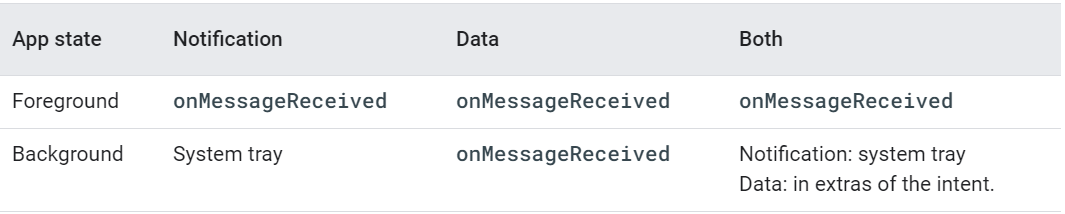
Handling Notification Payload:
处理通知有效负载:
When app is in background, notification is automatically handled by system and displayed without waking up the app.
当应用程序处于后台时,系统会自动处理通知并显示通知,而无需唤醒应用程序。
But for the foregrounded apps onMessageReceived function is to be called.
但是对于前台应用,应调用onMessageReceived函数。
How to call onMessageReceived ?
如何调用onMessageReceived?
We need to make an service extending FirebaseMessagingService.
我们需要提供扩展FirebaseMessagingService的服务。
Create a Java class MyFirebaseMessagingService extending FirebaseMessagingService.
创建Java类MyFirebaseMessagingService扩展FirebaseMessagingService。
Declare the activity as service in the App Manifest.
在应用清单中将活动声明为服务。
<service
android:name=".MyFirebaseMessagingService"
android:exported="false">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT"/>
</intent-filter>
</service>
In MyFirebaseMessagingService for the onMessageReceived function, write the following code:
在onMessageReceived函数的MyFirebaseMessagingService中,编写以下代码:
@Override
public void onMessageReceived(@NonNull RemoteMessage remoteMessage) {
super.onMessageReceived(remoteMessage);
sendNotification(remoteMessage);
}
private void sendNotification(RemoteMessage remoteMessage) {
Intent intent = new Intent(this, MainActivity.class);
PendingIntent pendingIntent =PendingIntent.getActivity(this,
m,intent,PendingIntent.FLAG_ONE_SHOT);
String channelId = getString(R.string.default_notification_channel_id);
Uri defaultSoundUri = RingtoneManager.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION);
NotificationCompat.Builder notificationBuilder;
notificationBuilder =
new NotificationCompat.Builder(this, channelId)
.setSmallIcon(R.drawable.geekhaven_transparent)
.setContentTitle(remoteMessage.getNotification.getTitle)
.setContentText(remoteMessage.getNotification.getBody)
.setAutoCancel(true)
.setSound(defaultSoundUri)
.setContentIntent(pendingIntent);
NotificationManager notificationManager =
(NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
// Since android Oreo notification channel is needed.
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
NotificationChannel channel = new NotificationChannel(channelId,
"Channel human readable title",
NotificationManager.IMPORTANCE_DEFAULT);
notificationManager.createNotificationChannel(channel);
}
notificationManager.notify(m, notificationBuilder.build());
If you are sending Only Notification Payload then the above method works. But if you want to send only Data Payload or both Data and Notification payload then you need to make a few changes:
如果要发送“仅通知有效负载”,则上述方法有效。 但是,如果您要仅发送数据有效载荷,或者仅发送数据有效载荷和通知有效载荷,则需要进行一些更改:
Change this
改变这个
.setContentTitle(remoteMessage.getNotification.getTitle)
.setContentText(remoteMessage.getNotification.getBody)
To
至
.setContentTitle(remoteMessage.getData.get("your_key1")
.setContentText(remoteMessage.getData.get("your_key2")
If you are using backend server to send notification, then you need to send the Registration Token to server every time it changes.
如果您使用后端服务器发送通知,则每次更改时都需要向服务器发送注册令牌。
For that you can look to onNewToken method in MyMessagingService
为此,您可以查看MyMessagingService中的 onNewToken方法
Here we will only focus on sending Manual Notifications. If you want to know about Backend server implementation you can have a look at Firebase Push Notifications To Android Using NodeJS.
在这里,我们仅专注于发送手动通知。 如果您想了解后端服务器的实现,可以看看Firebase使用NodeJS向Android推送通知 。
How to choose Payload?
如何选择有效载荷?
Choosing a right payload depends on your purpose. For example if you want to send only title and body as notification then go with Notification payload.
选择合适的有效负载取决于您的目的。 例如,如果您只想发送标题和正文作为通知,则可以使用Notification负载。
Otherwise if wish to send more data and even want to customize your Notification by adding actions, you should go with Only Data Payload as its always received in onMessageReceived method irrespective of the state of the app whereas for notification payload you can only customize it when app is in foreground because during background or killed state the notification is handled by system as it directly goes to the system tray.
否则,如果希望发送更多数据,甚至想要通过添加操作来自定义通知,则应将OnDataReceived方法中始终接收到的仅数据有效负载作为其始终接收,而与应用程序的状态无关,而对于通知有效负载,则只能在应用程序时自定义它之所以处于前台,是因为在后台或终止状态下,通知将直接进入系统托盘,由系统处理。
How to send FCM message manually?
如何手动发送FCM消息?
For sending FCM notification payload you can use Firebase Cloud Messaging Tool in firebase console.
要发送FCM通知有效负载,您可以在firebase控制台中使用Firebase Cloud Messaging Tool。
Go to Firebase Console — →Grow — →Cloud Messaging
转到Firebase控制台—→成长—→云消息传递
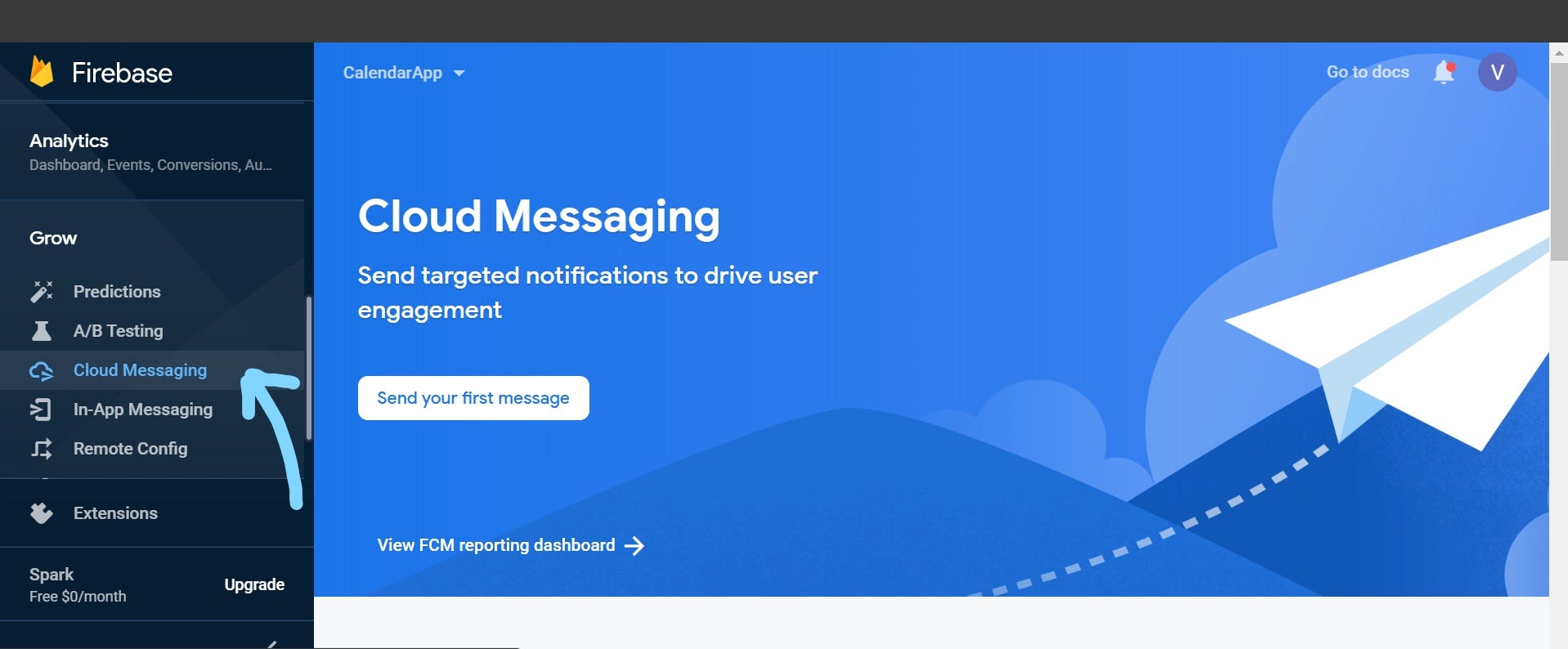
And click on Send your first message.
然后单击发送您的第一条消息。
Then enter the Title and body field.
然后输入标题和正文字段。
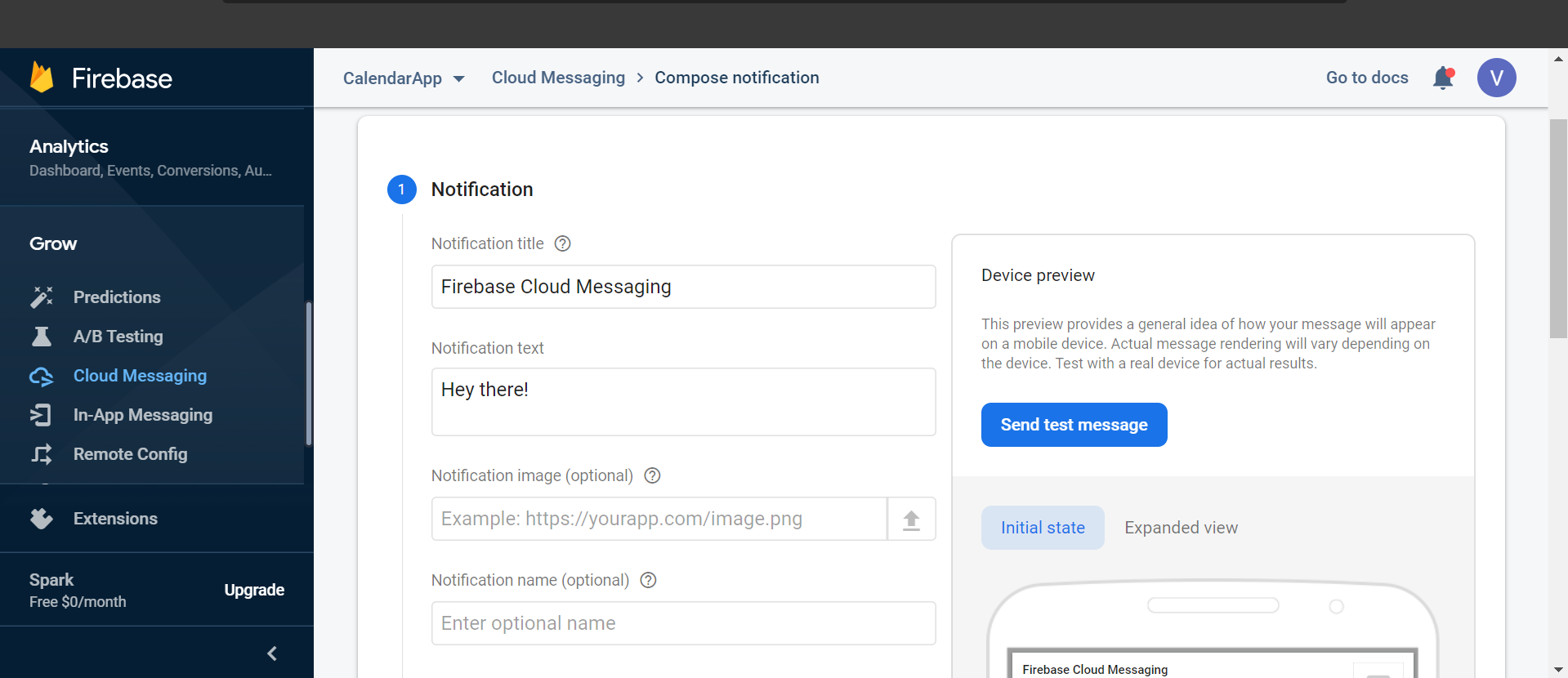
If you wish to send it to a particular device then click on Send test message and enter the FCM registration token.(Enter the current FCM registration token).
如果您希望将其发送到特定设备,请单击发送测试消息并输入FCM注册令牌。(输入当前的FCM注册令牌)。
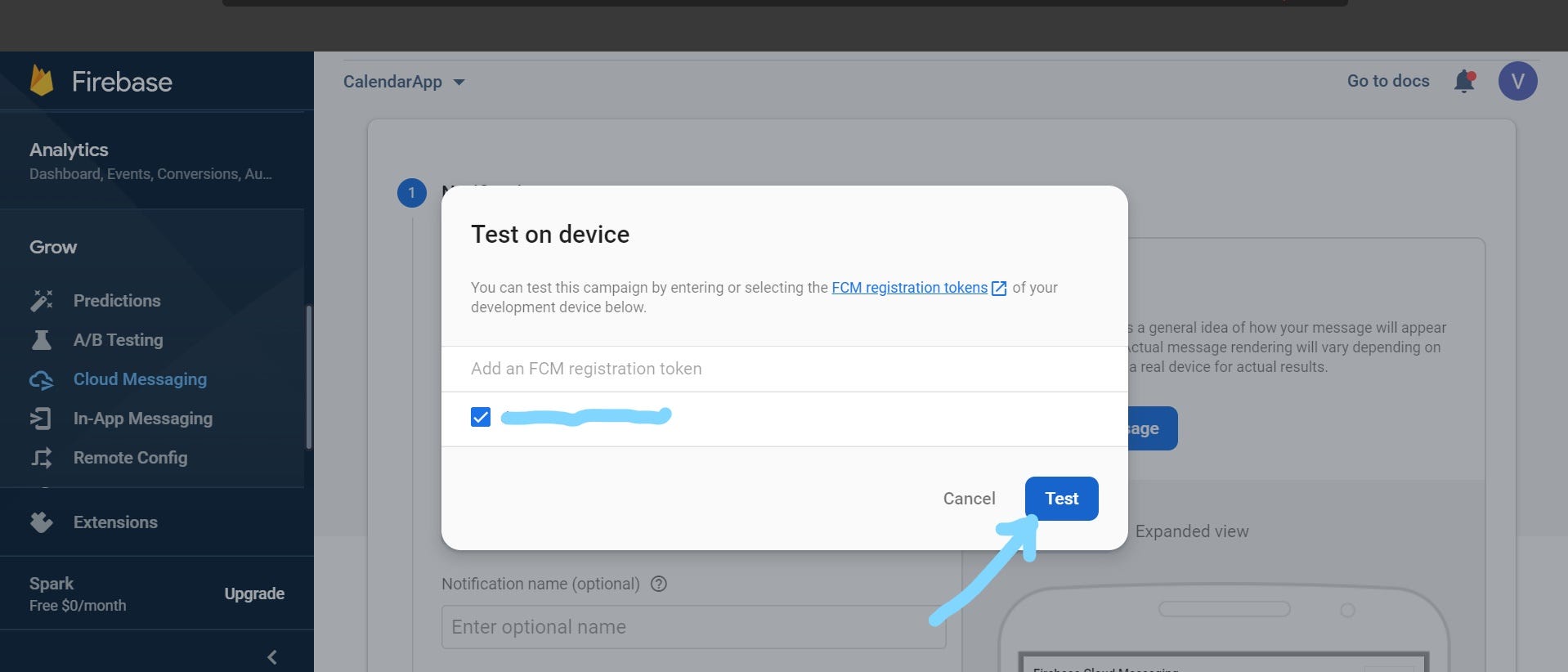
Click Test.
单击测试。
Whoa! you just received your FCM notification.
哇! 您刚刚收到了FCM通知。
Or
要么
If you want to send notification using Topic(discussed earlier) click on next ,select the current app and enter the topic name.
如果要使用主题(之前讨论过)发送通知,请单击下一步,选择当前应用程序并输入主题名称。
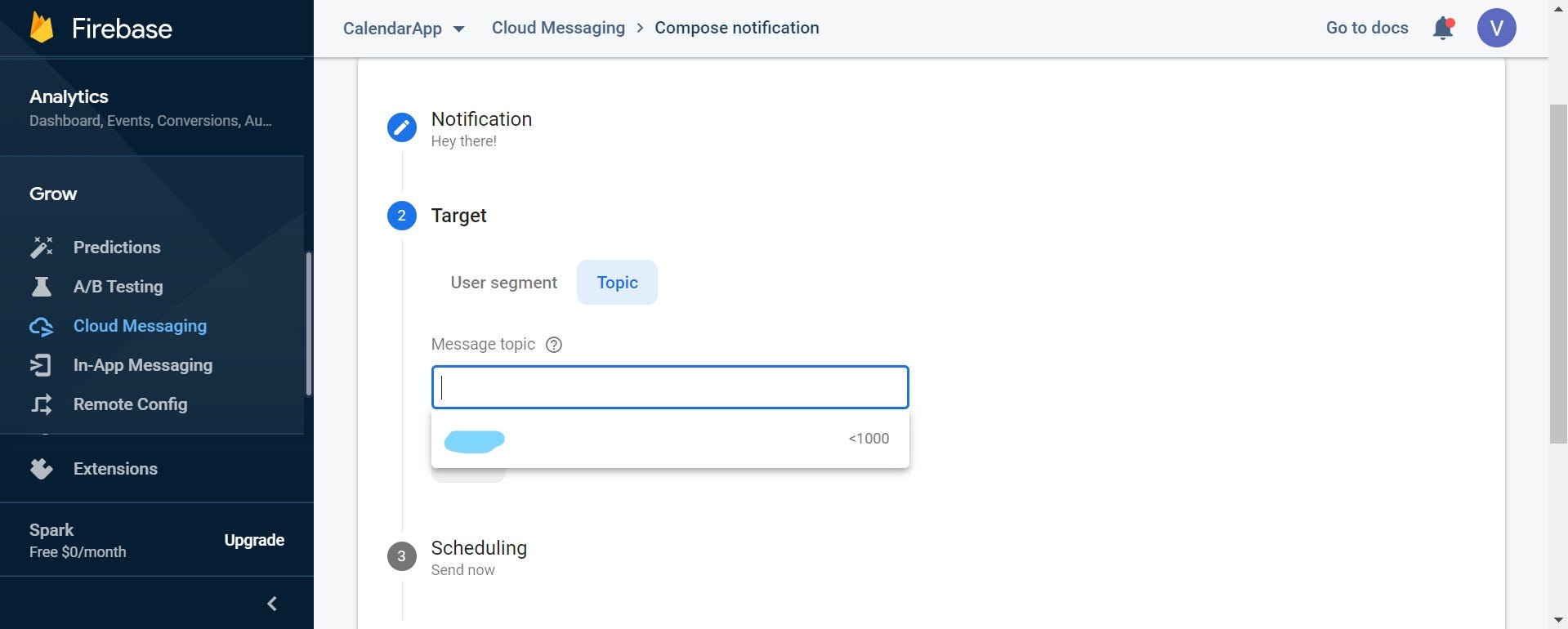
Click on publish!.
点击发布!
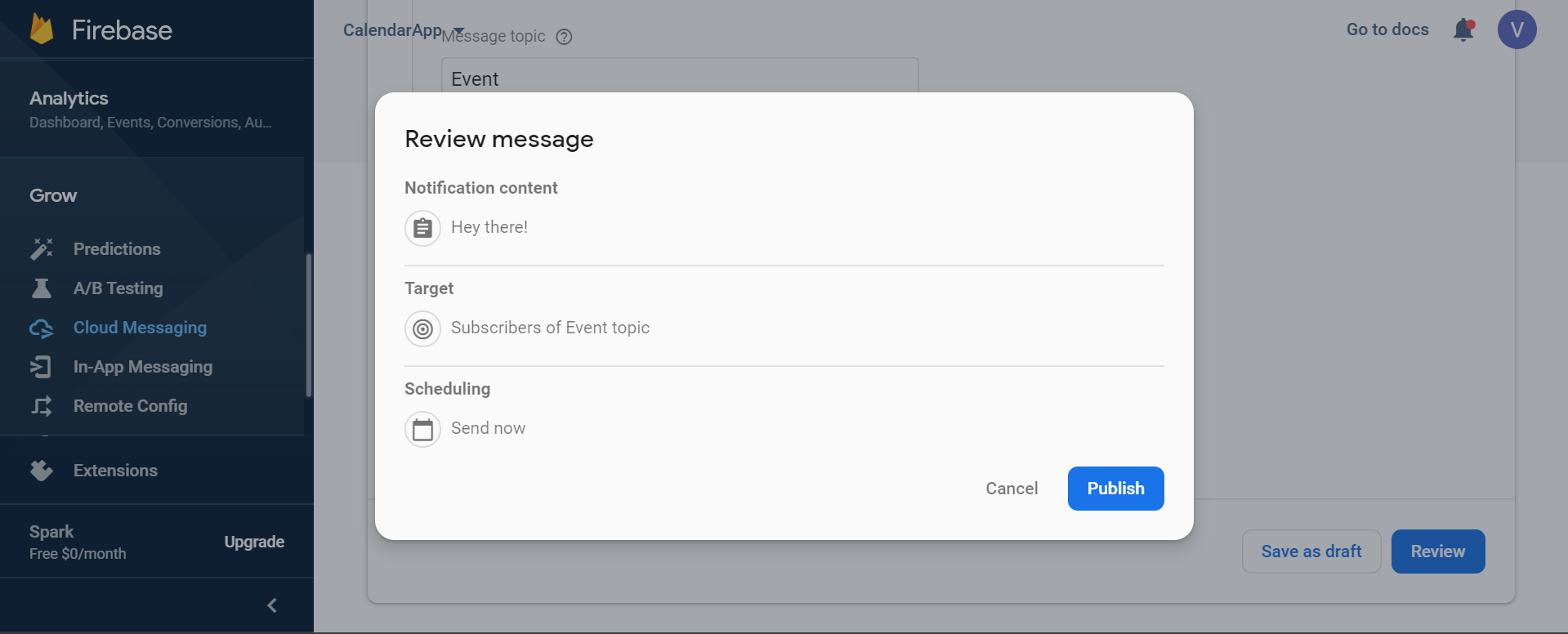
Whoa, here is the notification!
哇,这是通知!
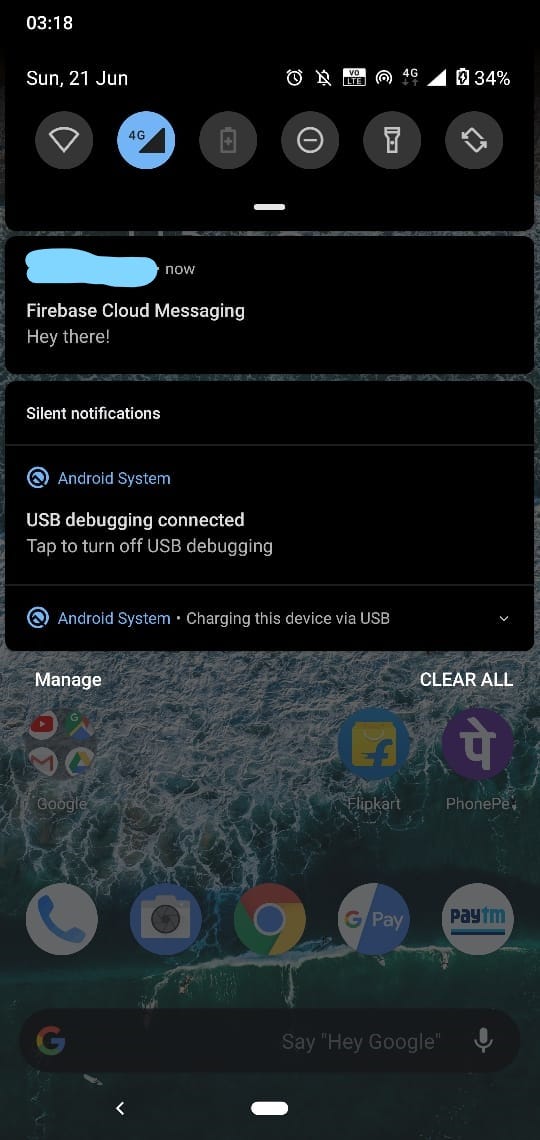
You may be wondering how could one send Data Payload messages or both Data and Notification payload manually. As of now there is no Firebase tool to send Data Payload messages, so we use POSTMAN.
您可能想知道一个人如何手动发送数据有效载荷消息或同时发送数据和通知有效载荷。 截至目前,还没有Firebase工具来发送数据有效载荷消息,因此我们使用POSTMAN。
Download POSTMAN application or add POSTMAN chrome extension.
下载POSTMAN应用程序或添加POSTMAN chrome扩展名。
Sending data message using HTTP protocol with POSTMAN:
使用HTTP协议和POSTMAN发送数据消息:
Step 1: Select POST . Enter request URL as https://fcm.googleapis.com/fcm/send
步骤1:选择POST。 输入请求网址为https://fcm.googleapis.com/fcm/send
Step 2: Add Headers Authorization: key=<server_key>
and Content-Type: application/json
.
步骤2:添加标头 Authorization: key=<server_key>
和Content-Type: application/json
。
How to get Server_key?
如何获得Server_key?
Go to Firebase console — →Project Settings — →Cloud Messaging.
转到Firebase控制台—→项目设置—→云消息。
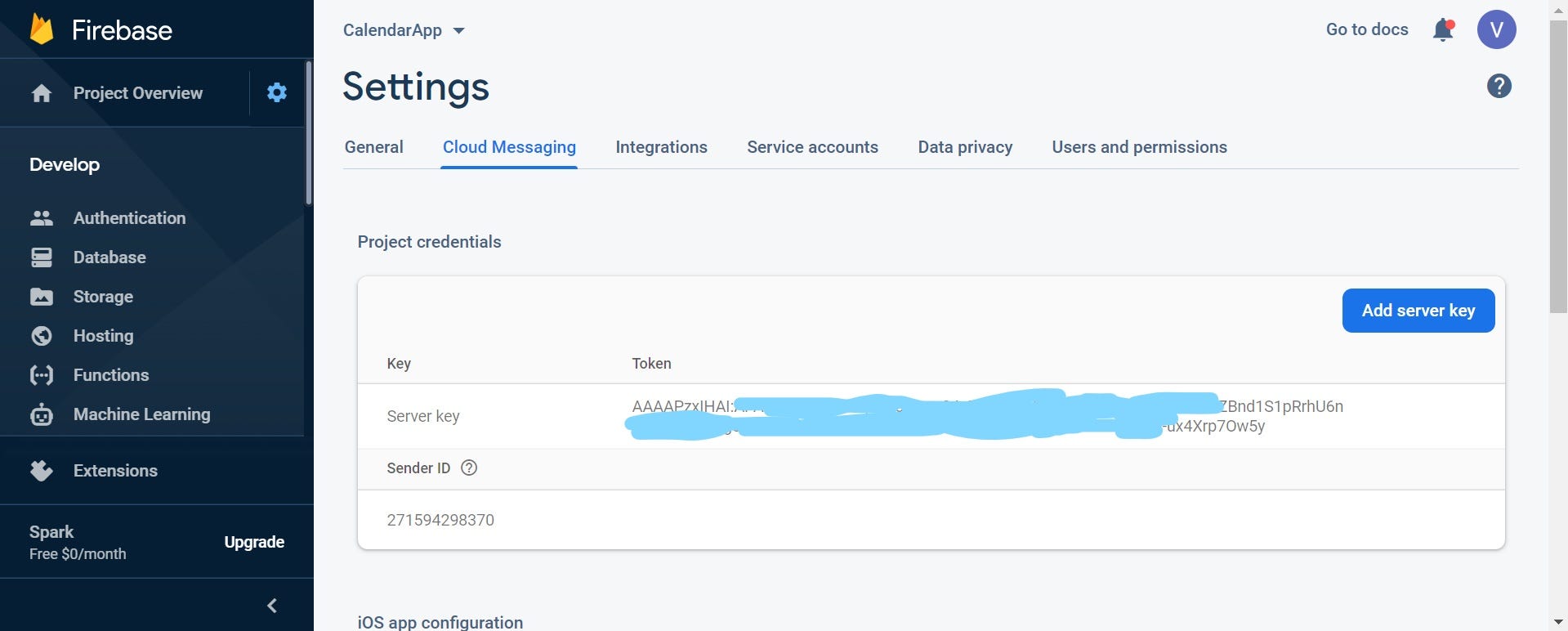
To send the message select Body — →Raw — →JSON(application/json).
要发送消息,请选择正文—→原始—→JSON(application / json)。
{
"to" : "YOUR_FCM_TOKEN",
"data" : {
"body" : "Notification Body",
"title": "Notification Title",
"key_1" : "Value for key_1",
"key_2" : "Value for key_2"
}
}
You can send Notification Payload , Data Payload and even both using POSTMAN service.
您可以使用POSTMAN服务发送Notification Payload,Data Payload,甚至都发送。
{
"to" : "YOUR_FCM_TOKEN",
"notification" : {
"body" : "Body of Your Notification",
"title": "Title of Your Notification"
},
"data" : {
"body" : "Notification Body",
"title": "Notification Title",
"key_1" : "Value for key_1",
"key_2" : "Value for key_2"
}
}
To send Notification to more than one device use registration_ids
instead of to
or use "to":"topic/topic_name"
.
要将Notification发送到多个设备,请使用registration_ids
而不是to
或使用"to":" topic/topic_name "
。
About me
关于我
I am a Tech enthusiast and second year B.Tech IT student at IIIT Allahabad who is always curious about Android related stuff!
我是一名技术爱好者,也是IIIT Allahabad的B.Tech IT大学二年级学生,他一直对Android相关知识感到好奇!
翻译自: https://medium.com/nybles/sending-push-notifications-by-using-firebase-cloud-messaging-249aa34f4f4c
firebase 推送